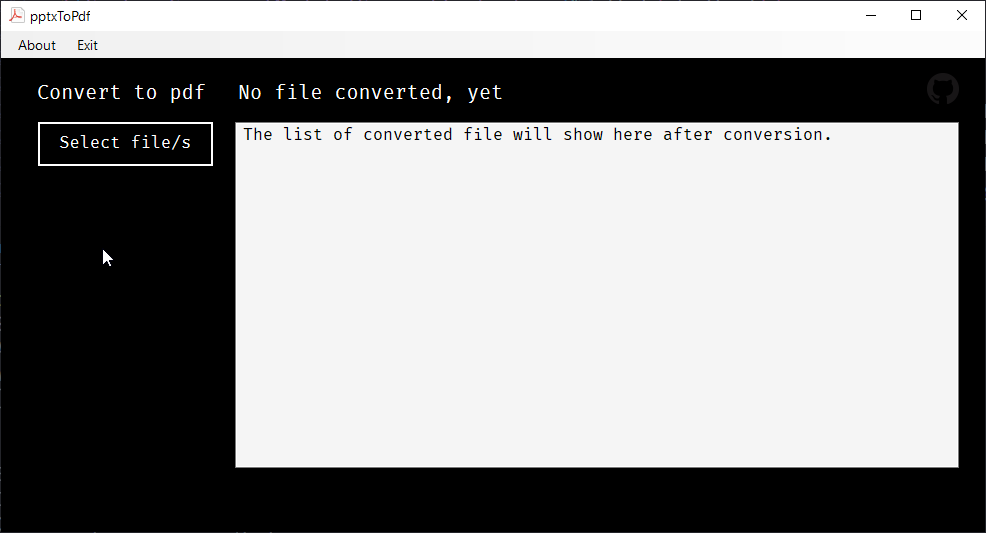
Since at work we use windows systems, I had to go through the path of Powershell for system automation, and while I hated it at first, my interest slowly grown when I realized I could use .NET modules such as emails and others without having to reinvent the wheel, so here I am presenting a super simple GUI app which will do only one thing: convert Powerpoint files to pdf in bulk.
I originally thought this small project as a CLI script, but I wanted to see what it feels like creating a minimal app using windows forms.
I need to to be honest, design a windows form without a "form design" tool was a real pain, as I had to look up each component I wanted, also the code was really repetitive and placing objects on the form was a mess since you don't see them until you run the script, positioning is a real challenge.
So, I decided to create a Powershell Windows form project in Visual Studio Community 2022, as it as all the feature to design forms.
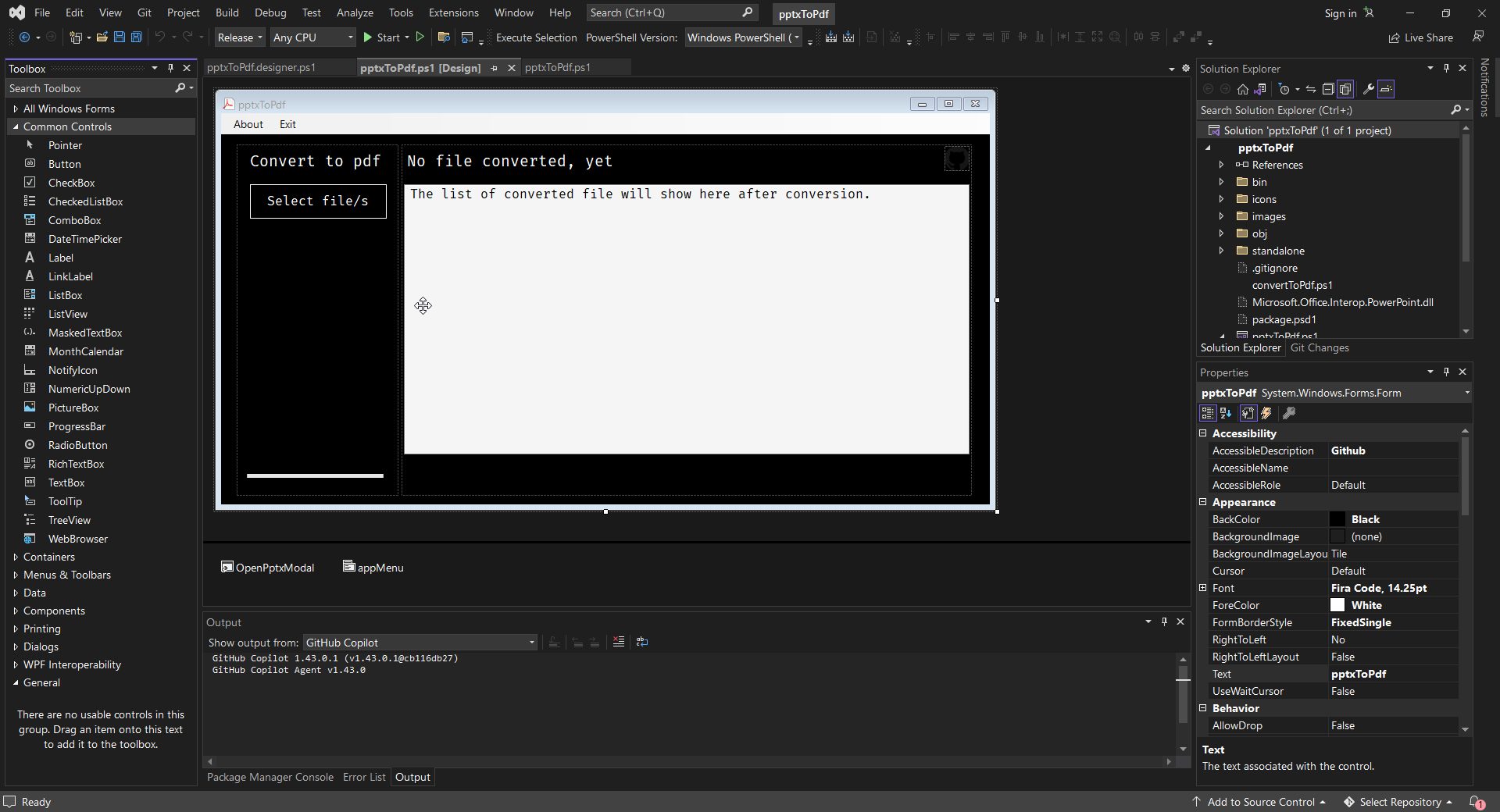
This greatly increased the speed of the project as all I had to do to initialize a form component was drag and drop into the app window and change whatever options on the bottom right panel, this will create the form controls code on his own, which is way better then typing all the repetitive controls yourself.
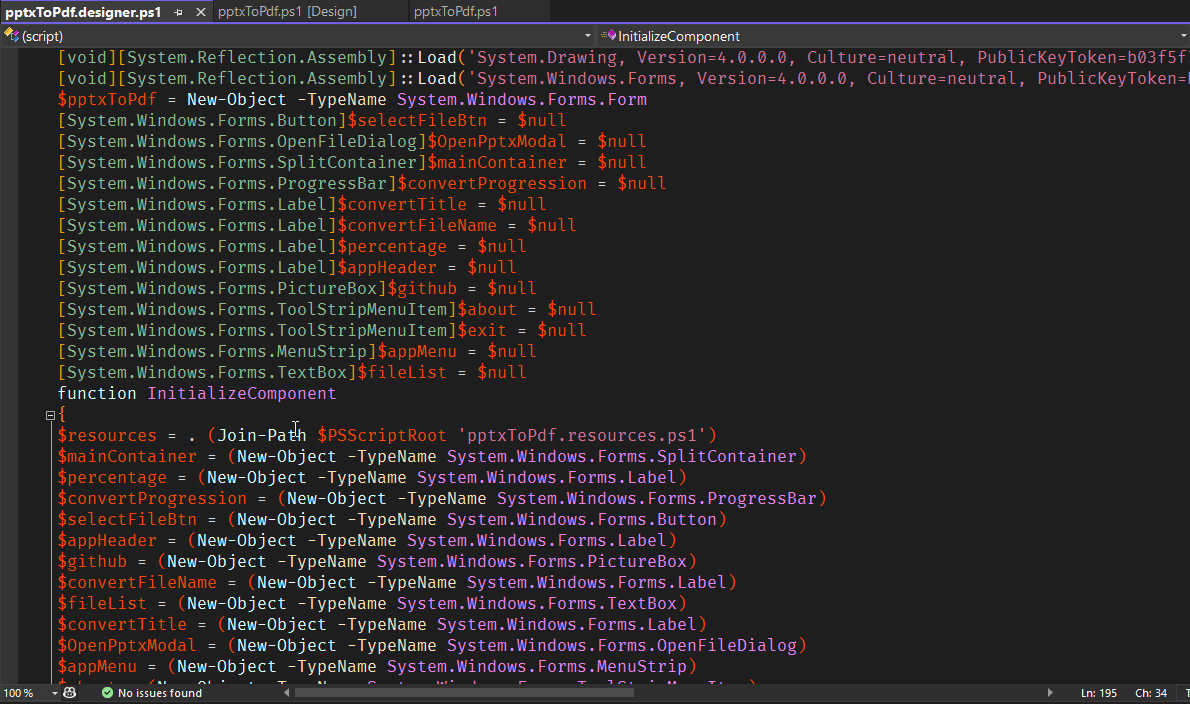
This app was inspired by this blog post: Updated Events GitHub repository – Convert pptx to pdf
where he does show how to get powerpoint files from a folder and then convert them to a pdf. I had to modify the script a tiny bit for my needs, then on top of it I did add a Powershell GUI using Windows forms.
Note that to run this app you need:
The app itself is very simple, it only use few controls:
- SplitContainer - handle the layout columns
- Button - open OpenFileDialogue
- OpenFileDialogue - to pick your *.pptx files
- ProgressBar - show the conversion progress
- Labels - simple inputs text
- PictureBox - hold a link to the Github repo
- MenuStrip - contains all the menu elements
- ToolStripMenuItem - has the menu items
- TextBox - list of converted files
ConvertToPdf.ps1
This ps1 file has a couple of functions:
- DisplayFiles
- ConvertToPdf
DisplayFiles, just do what his name implies, it prints out the name of the converted files. They're saved inside an array. It also hide few controls we don't want to see at this time.
ConvertToPdf, runs on a click event listener, which opens up a OpenFileDialogue, if you correctly select some *pptx files, it will return a "OK" response and then convert.
While processing the files it will show a progressbar with a percentage and the current file name.
To convert the file we use the Microsoft Assembly: Microsoft.Office.Interop.PowerPoint.dll
, you can download it with Nuget package manager: Microsoft.Office.Interop.PowerPoint
. (Join-Path $PSScriptRoot 'pptxToPdf.designer.ps1')
function DisplayFiles {
Param(
[Parameter(Mandatory=$true,Position=0)]
[System.Object]$modalTitle
)
$convertFileName.Text = ""
$convertProgression.Visible = $false
$percentage.Visible = $false
$files = $modalTitle.FileNames
$arr = @()
foreach($file in $files) {
$fileName = Split-Path $file -Leaf
$arr += $fileName
$fileList.Lines = $arr
}
if($files.Count -gt 1) {
$convertTitle.Text = "Converted files: $($files.count)"
}
else {
$convertTitle.Text = "Converted file: $($files.count)"
}
}
function ConvertToPdf {
Param(
[Parameter(Mandatory=$true,Position=0)]
[System.Object]$modalTitle
)
$selectedFiles = $modalTitle.ShowDialog()
if($selectedFiles -eq "OK") {
$files = $modalTitle.FileNames
foreach ( $file in $files ) {
# ProgressBar
$convertProgression.Visible = $true
$percentage.Visible = $true
$i++
[int]$progress = ($i / $files.count) * 100
$convertProgression.Value = $progress
$percentage.Text = "$progress%"
$convertProgression.Update()
$fileName = Split-Path $file -Leaf
$convertFileName.Text = "Converting: $fileName"
# End ProgressBar
Get-ChildItem $file -File -Filter *pptx -Recurse |
ForEach-Object -Begin {
$null = Add-Type -Path $PSScriptRoot\Microsoft.Office.Interop.PowerPoint.dll
$SaveOption = [Microsoft.Office.Interop.PowerPoint.PpSaveAsFileType]::ppSaveAsPDF
$PowerPoint = New-Object -ComObject "PowerPoint.Application"
} -Process {
try {
$Presentation = $PowerPoint.Presentations.Open($_.FullName)
$PdfNewName = $_.FullName -replace '\.pptx$','.pdf'
$Presentation.SaveAs($PdfNewName,$SaveOption)
$Presentation.Close()
}
catch {
Write-Error $_ -ErrorAction SilentlyContinue
}
} -End {
$PowerPoint.Quit()
Stop-Process -Name POWERPNT -Force
}
}
DisplayFiles $modalTitle
}
else {}
}
function ShowAbout {
[void] [System.Windows.Forms.MessageBox]::Show(
" pptxToPdf v1.0.0`n`n Author: Paniconi Fabio`n`n Email: [email protected]`n`n Repo: https://github.com/Dragod/bulk-pptx-to-pdf `n`nThis script is free software: you can redistribute it and/or modify`n it under the terms of the GNU General Public License as published by`n the Free Software Foundation, either version 3 of the License, or`n (at your option) any later version.`n`nThis program is distributed in the hope that it will be useful,`n but WITHOUT ANY WARRANTY; without even the implied warranty of`n MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the`n GNU General Public License for more details.`n`nYou should have received a copy of the GNU General Public License`n along with this program. If not, see <http://www.gnu.org/licenses/>.",
"About pptxToPdf",
"OK",
"Information"
)
}
pptxTopdf.ps1
This script is very simple, it imports other ps1 files and then add click events and show the app GUI at the end, when $pptxToPdf.ShowDialog()
is called.
. (Join-Path $PSScriptRoot 'pptxToPdf.designer.ps1')
. (Join-Path $PSScriptRoot 'convertToPdf.ps1')
[System.Windows.Forms.Application]::EnableVisualStyles()
$about.Add_Click({ShowAbout})
$exit.Add_Click({$pptxToPdf.Close()})
$github.Add_Click({ Start-Process 'https://github.com/Dragod/bulk-pptx-to-pdf' }.GetNewClosure())
$selectFileBtn.Add_Click({ConvertToPdf $OpenPptxModal})
$pptxToPdf.ShowDialog()
$pptxToPdf.Dispose()
Repository
Github repository: pptxToPdf