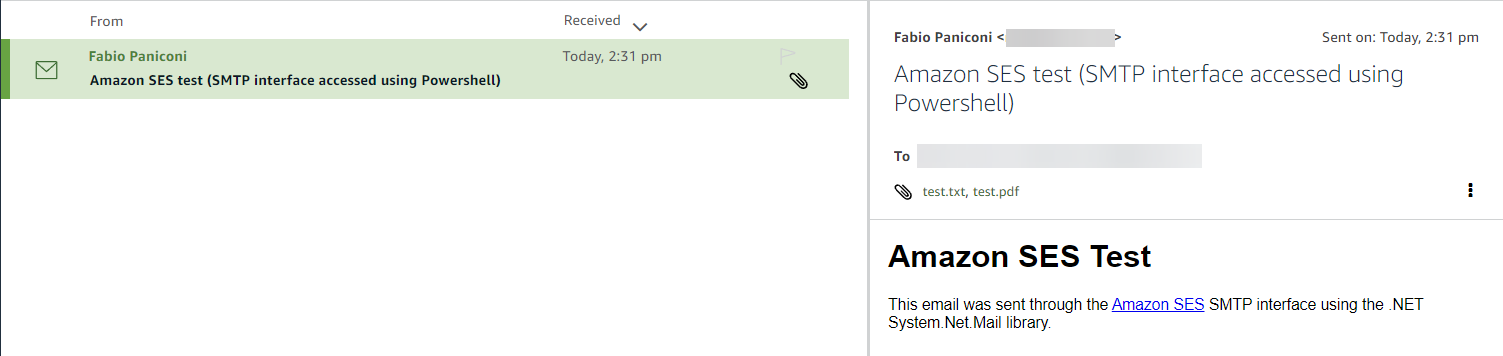
I have seen plenty of blog posts explaining how to do this with C# but none using Powershell.
There isn't a big difference to be honest, but it's a small nice script to send automated emails if you are a system administrator.
Of course, this could be improved but I just wanted to show the basic functionality.
Config.json
The config.json
will just hold all the configuration needed to send the email.
{
"smtp":
{
"server": "smtp_server_here",
"port": 587,
"user": "user_here",
"password": "password_here"
},
"headers":
{
"ses": "config_set_here"
},
"email":
{
"from": "yourEmail.com",
"to": "[email protected],[email protected]",
"subject": "Amazon SES test (SMTP interface accessed using Powershell)",
"body": "<h1>Amazon SES Test</h1> <p>This email was sent through the <a href='https://aws.amazon.com/ses'>Amazon SES</a> SMTP interface using the .NET System.Net.Mail library.</p>",
"isHtml": true,
"attachments": [
{
"path": "test.txt",
"mimeType": "plain/text"
},
{
"path": "test.pdf",
"mimeType": "application/pdf"
}
]
}
}
Mail.ps1
Setup the email object we want to send.
- Email from
- Email to
- Email Subject
- Email body
- Email attachment/s
Also you can specify other things such as:
- If you want the email to contains plain text or HTML
- Sending Email headers
$config = Get-Content -Path "$PSScriptRoot\config.json" | ConvertFrom-Json
# Set up the mail message
$email = New-Object System.Net.Mail.MailMessage
Write-Host "`nServer: $($config.smtp.server)" -ForegroundColor Magenta
$email.Headers.Add("X-SES-CONFIGURATION-SET", $config.headers.ses)
Write-Host("`nEmail from: " + $config.email.from) -ForegroundColor Magenta
$email.From = $config.email.from
$to = @($config.email.to).split(",").trim()
Write-Host("`nEmail to: " + $config.email.to) -ForegroundColor Magenta
foreach ($addr in $to)
{
$email.To.Add($addr)
}
$email.Subject = $config.email.subject
Write-Host("`nSubject: " + $config.email.subject) -ForegroundColor Magenta
$email.IsBodyHtml = $config.email.isHtml
$email.Body = $config.email.body
foreach ($attachment in $config.email.attachments) {
Write-Host "`nAttachment:" $attachment.path -ForegroundColor Magenta
$attachment = New-Object System.Net.Mail.Attachment("$PSScriptRoot\$($attachment.path)", $attachment.mimeType)
$email.Attachments.Add($attachment)
}
Send.ps1
We can finally send our email using send.ps1
.
We import the other ps1 scripts, then create the .Net Mail object and send the email.
. (Join-Path $PSScriptRoot 'mail.ps1')
$config = Get-Content -Path "$PSScriptRoot\config.json" | ConvertFrom-Json
$smtpClient = New-Object System.Net.Mail.SmtpClient($config.smtp.server , $config.smtp.port)
$smtpClient.EnableSsl = $true
$smtpClient.Credentials = New-Object System.Net.NetworkCredential($config.smtp.user , $config.smtp.password)
try {
Write-Host("`nAttempting to send email...") -ForegroundColor Yellow
$smtpClient.Send($email)
Write-Host("`nEmail sent!`n") -ForegroundColor Green
}
catch {
Write-Host("`nThe email was not sent.")
Write-Host("`nError sending email: $_")
}
Sending the email
Open Powershell CLI, them make sure you are in the root folder of your project
.\send.ps1
Server: your.smtp.server
Email from: yourEmail.com
Email to: [email protected],[email protected]
Subject: Amazon SES test (SMTP interface accessed using Powershell)
Attachment: test.txt
Attachment: test.pdf
Attempting to send email...
Email sent!